We did this using a little javascript, a scheduled github action workflow and a click-opsed slack webhook.
This is what the action ended up looking like. A schedule each monday morning UTC, that checks out the repo, sets up node, and calls an npm script.
name: post opser to slack
on:
workflow_dispatch:
schedule:
- cron: '0 7 * * 1'
jobs:
post-to-slack:
runs-on: ubuntu-latest
steps:
- name: checkout code
uses: actions/checkout@v3
- name: setup node
uses: actions/setup-node@v3
with:
node-version: 18.17.1
- name: run script
run: npm run post-opser-to-slack
The javascript behind the script post-opser-to-slack
looked like this. It cycles between the list of team member emails, and posts one to slack each week. ChatGPT helped getting the current iso week number from a javascript Date
.
(async function () {
var webHookUrl = "https://hooks.slack.com/triggers/a/b/c";
// credits chatgpt
function isoWeek(date) {
// copy date so don't modify original
date = new Date(date);
// set to nearest thursday (current date + 4 - current day) % 7
date.setUTCDate(date.getUTCDate() + 4 - (date.getUTCDay() || 7));
// get first day of year
var yearStart = new Date(Date.UTC(date.getUTCFullYear(), 0, 1));
// calculate full weeks to nearest thursday
var week = Math.ceil(((date - yearStart) / 86400000 + 1) / 7);
return week;
}
// fill this with team member slack email adresses
var team = [];
var memberByWeek = [...Array(53).keys()]
.map((n) => n + 1)
.map((week) => team[(week - 1) % team.length]);
var member = memberByWeek[isoWeek(new Date())];
await fetch(webHookUrl, {
method: "POST",
body: JSON.stringify({ member }),
headers: {
"content-type": "application/json",
},
});
})();
Yes, some years actually have 53 weeks.
An ISO week-numbering year (also called ISO year informally) has 52 or 53 full weeks. That is 364 or 371 days instead of the usual 365 or 366 days. These 53 week years occur on all years that have Thursday as the 1st of January and on leap years that start on Wednesday the 1st. The extra week is sometimes referred to as a leap week, although ISO 8601 does not use this term.
In a paid slack organization you can create a webhook workflow clicking More -> Automations -> + -> Workflow Builder -> From a webhook
, and set a key called member
with the data type of Slack user email
.
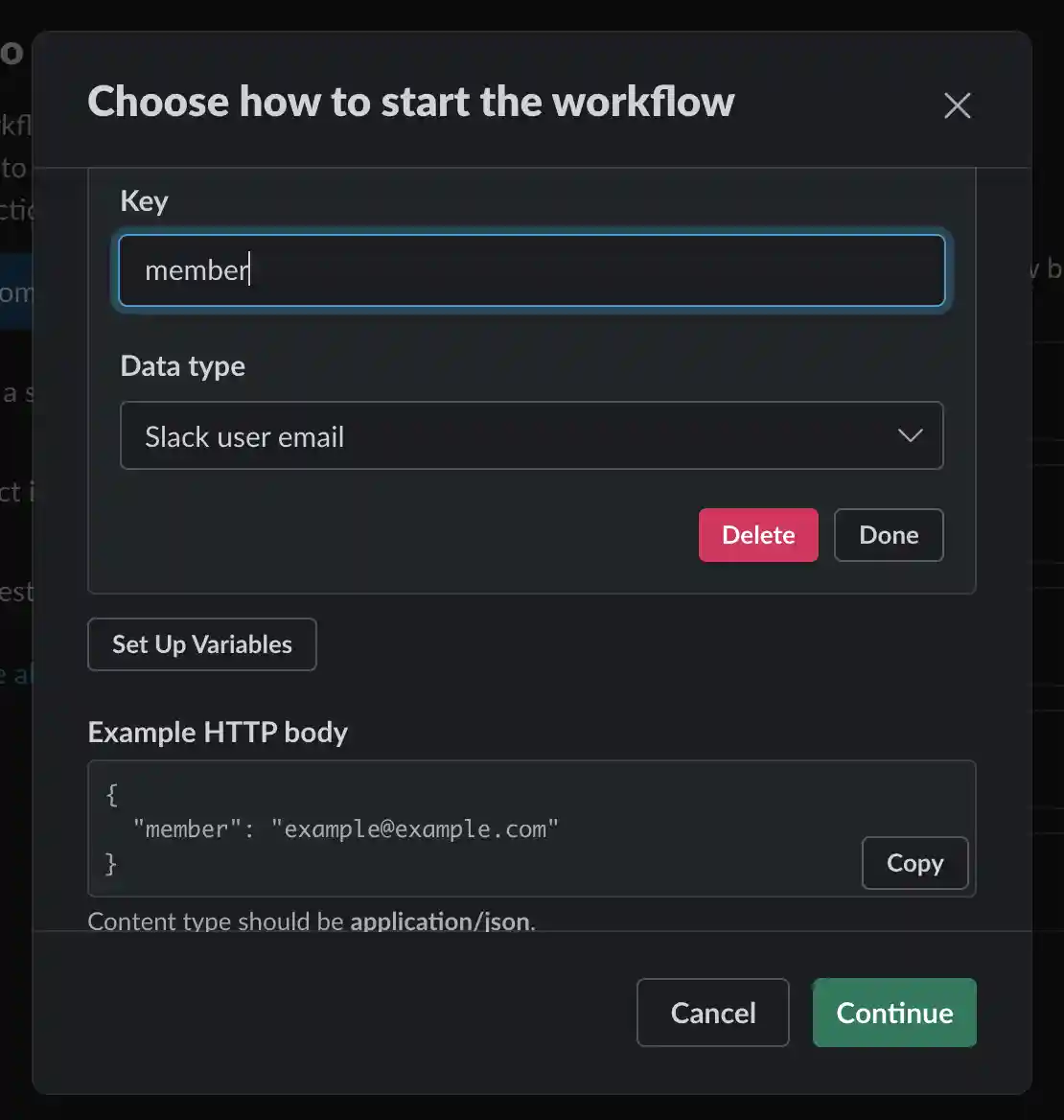
Connect it to an action, e.g. Messages -> Send message to a channel
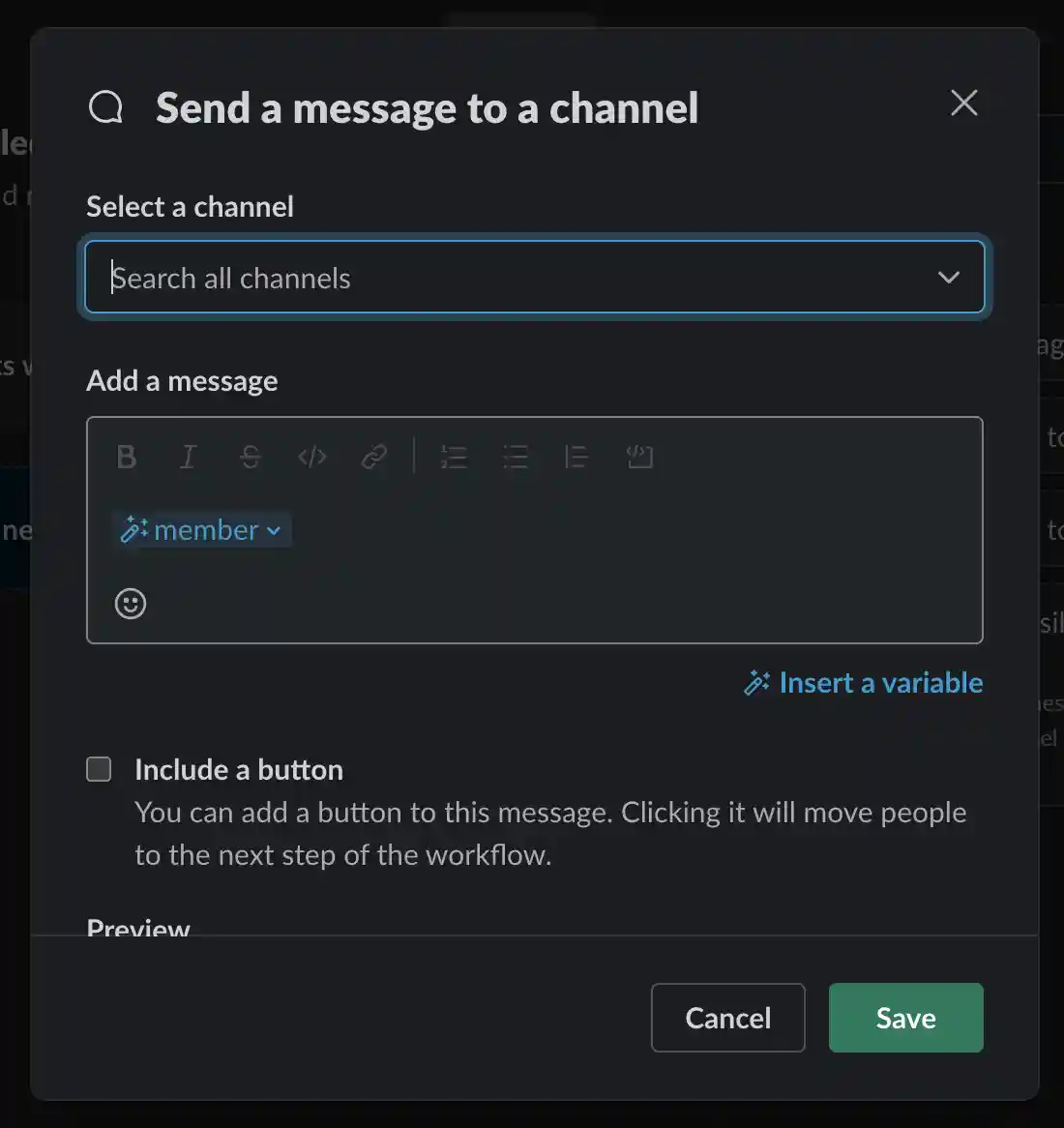
Update the javascript above with the url of the webhook, and wait for your turn! ↩️
I love these kinds of low key automations that removes manual labor and lets us focus on the important stuff 😍